Do you know about the PARAMS keyword in C# ?
The params keyword allows us to create methods that accept a variable number of arguments of the same type.
The params parameter is always an array, can only be used once and it must be the last parameter in the list of parameters.
Here’s a simple example where we sum numbers together :
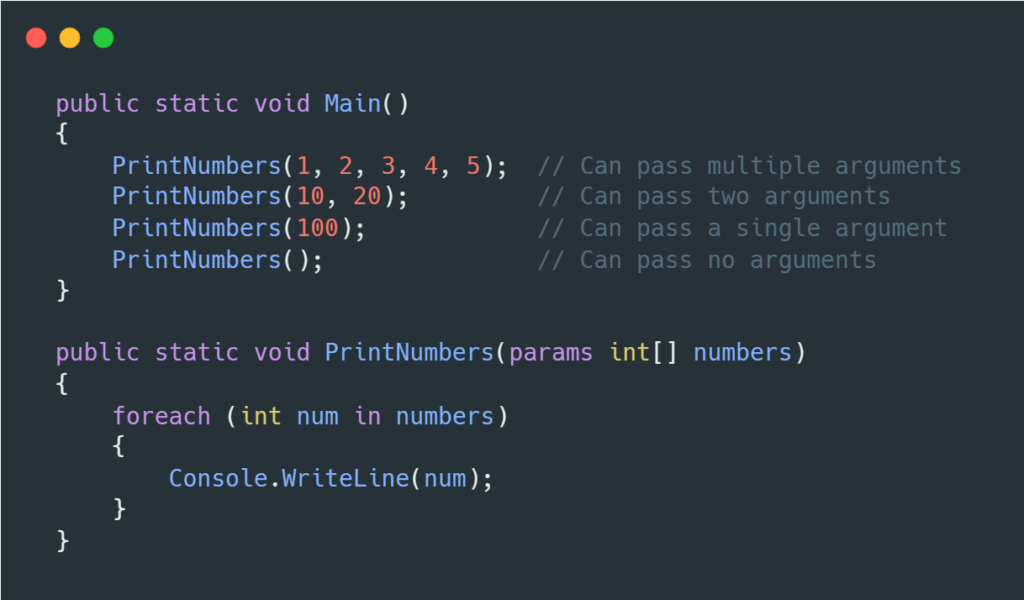
By using params we don’t need to provide explicit overloads for each different amount of numbers.
We could of course also pass an array explicitly if we wish.
For example …
PrintNumbers(new int[] {1, 2, 3});
… but the main advantage of params
is that you don’t have to create the array manually each time you call the method.
Here’s two more examples using a normal and a jagged array …
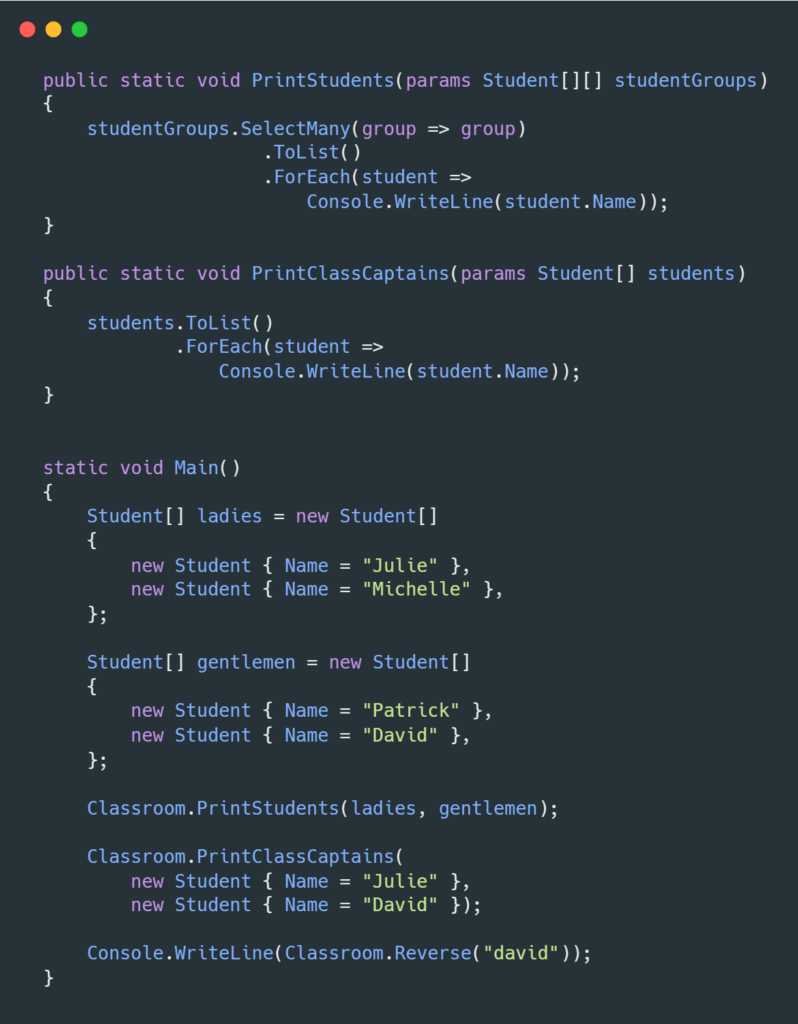
Params can also be used in combination with generics :
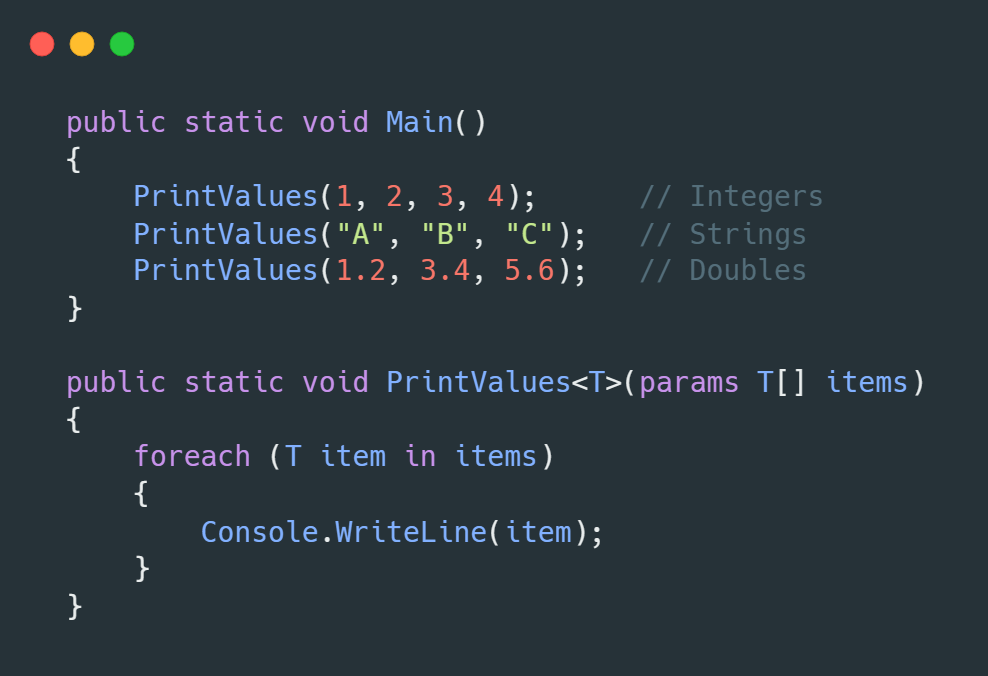
We can also apply constraints to the generic type when using params :
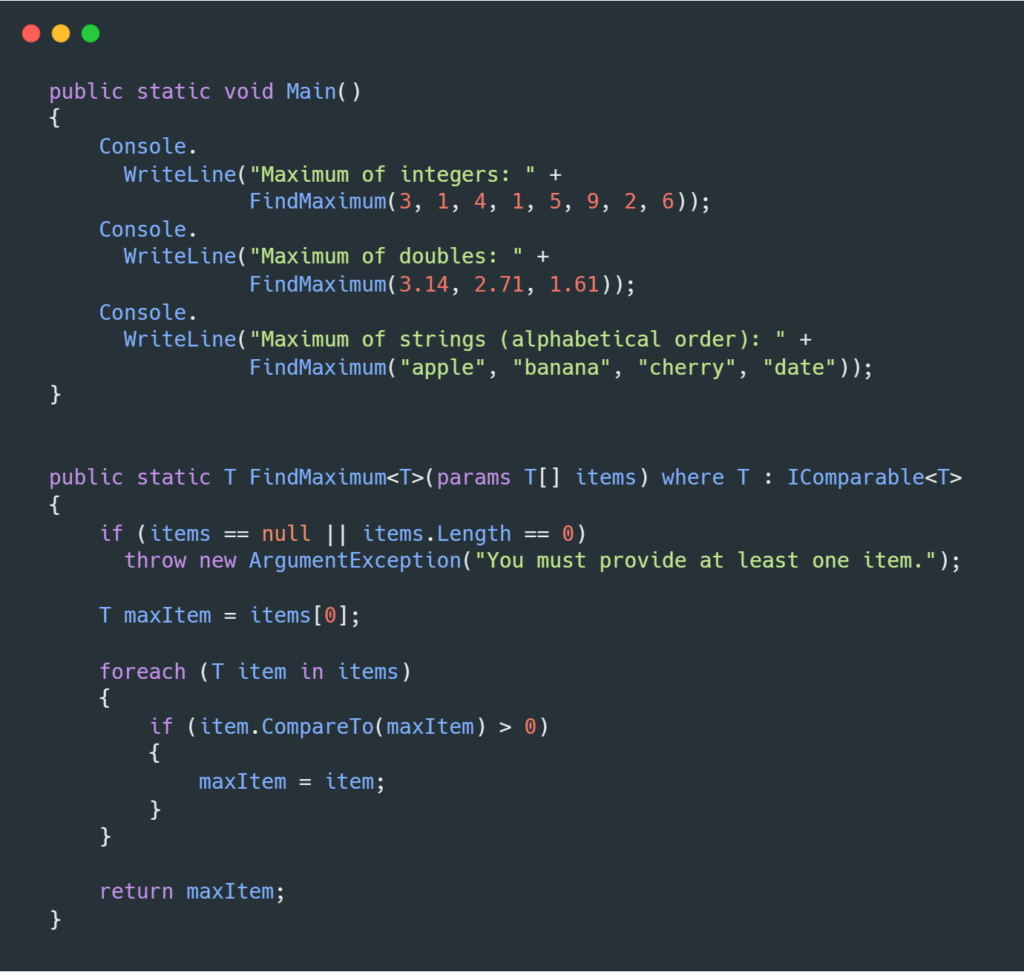
Performance implications?
There’s a performance overhead to using params versus explicitly creating overloads for different amounts of arguments and this is why in the .NET framework itself there are often explicit overloads as well as a params one.
This is entirely appropriate for a framework but the performance difference isn’t likely to matter to most apps as depending on what you’re doing we could be talking about nanoseconds so go ahead and use params. Its very helpful to give our methods a lot more flexibility.
Here’s an example where I’m concatenating three strings with the benchmarked methods below …
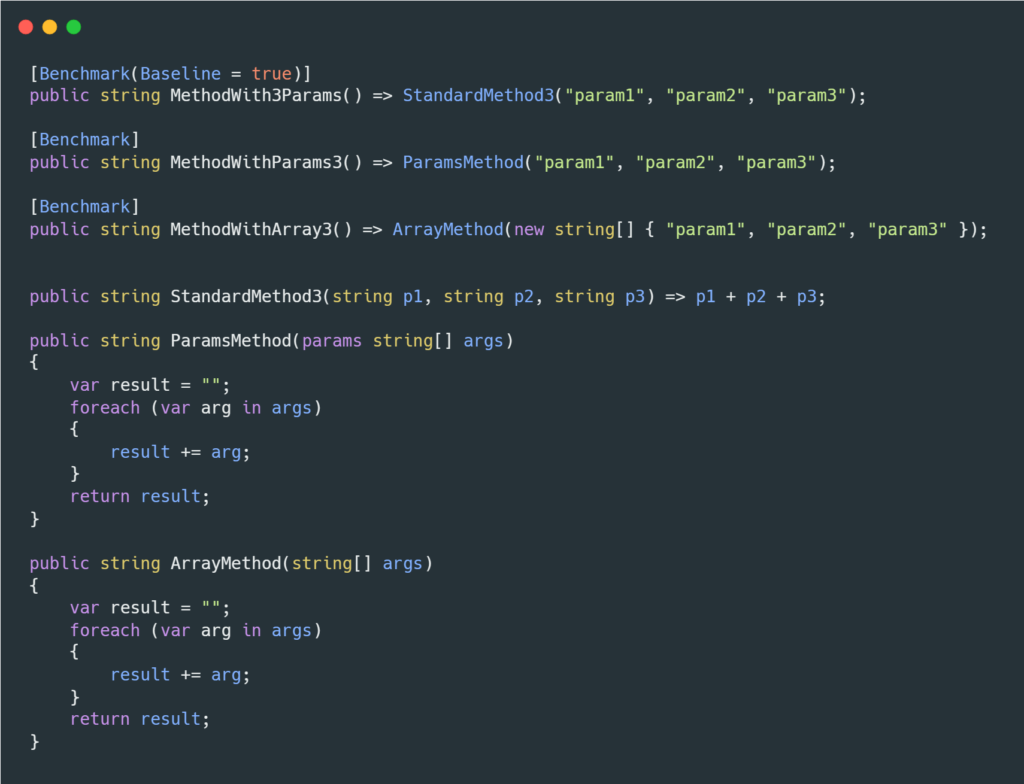
We can see from the results below that while relatively speaking the Params approach (and array approach for which Params is essentially shorthand for) is 105% slower, in absolute terms the difference is only 15 or 16 nanoseconds (for this case) …
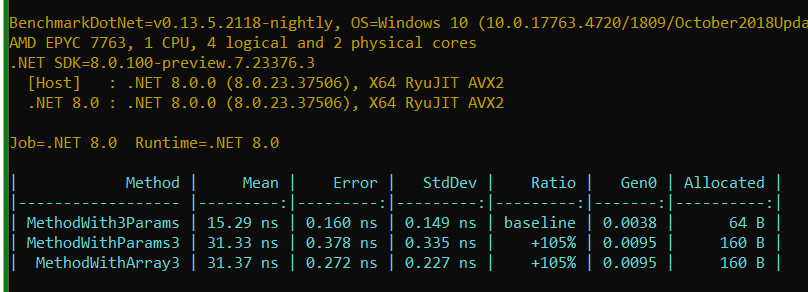
Obviously this difference is just for this particular case and for the particular run shown above but in return for the small performance hit we don’t have to define lots of explicit overloads for 2 params, 3 params, 4 params etc.
BUT … as always, check your own specific case.
Some Params keyword usages in .NET
The .NET runtime itself uses params a good bit.
The below usages are on GitHub and you need to be signed in.
Params string[] usages in .NET ->
Code search results (github.com)
Params int[] usages in .NET ->
Code search results (github.com)
Params T usages in .NET ->
Code search results (github.com)
What do you think?
Have you used params in your methods before?
Grab Your Free C# Cheat Sheet!
Download "C# Essentials: A Developer's Cheat Sheet" for key syntax, tips, and quick references. Perfect for developers of all levels!
👉 Download your free copy now!Need Help with Your C# Projects?
We offer expert support and development services for projects of any size. Contact us for a free consultation and see how we can help you succeed.
CONTACT US NOW